Map, Reduce and Filter in JavaScript
Map, Reduce and Filter are three declarative and higher-order array methods that are particularly important in JavaScript applications and even the majority of all modern programming languages. Each of these three methods iterates over a given array and performs specific actions. Steven Luscher humorously described them in a single tweet, as shown below.
Map/filter/reduce in a tweet:
— Steven Luscher (@steveluscher) June 10, 2016
map([🌽, 🐮, 🐔], cook)
=> [🍿, 🍔, 🍳]
filter([🍿, 🍔, 🍳], isVegetarian)
=> [🍿, 🍳]
reduce([🍿, 🍳], eat)
=> 💩
The map Method
The map(callback) method returns a new array by applying the given callback function to every element of the given array. Let’s initially declare an array of books, where each book is an object, as follows:
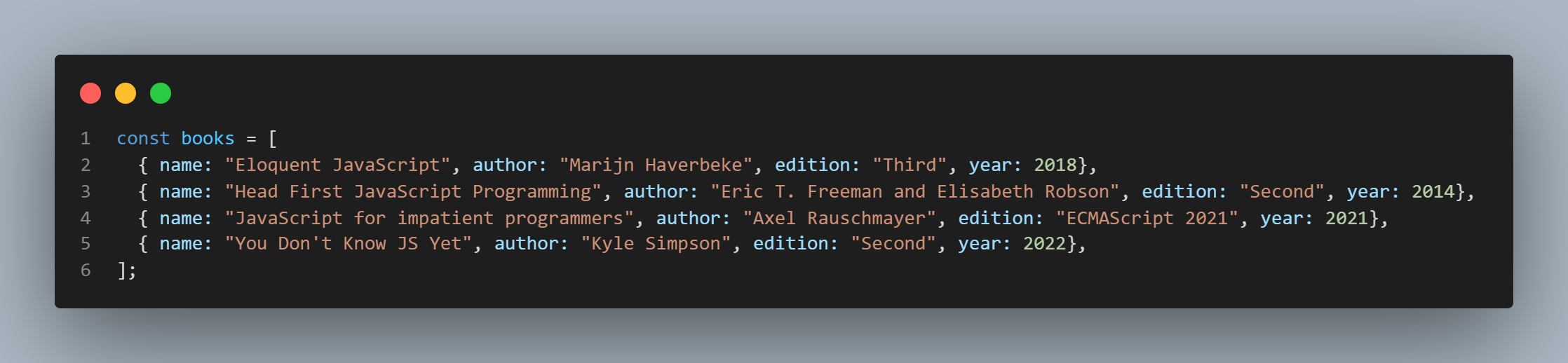
Now, let’s see how to use the map() method to map the details of each book into a sentence.
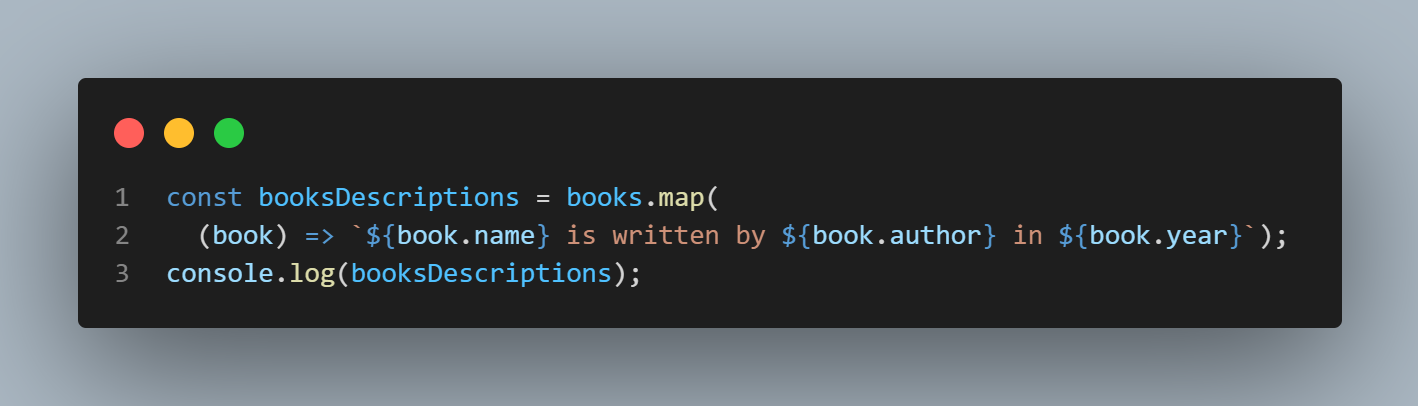
Remember that the map method returns a new array, so it is an anti-pattern not to use the returned array. Therefore, it would be better to use the forEach or for…of loops if you are not going to use the returned array or if the used callback does not return a value.
The filter Method
The filter(callback) method creates a new array with all elements that pass the condition made by the given callback function. The filter() method would return an empty array if all elements did not pass the condition. The following code snippet uses the filter() method to return books that have been published after 2018.
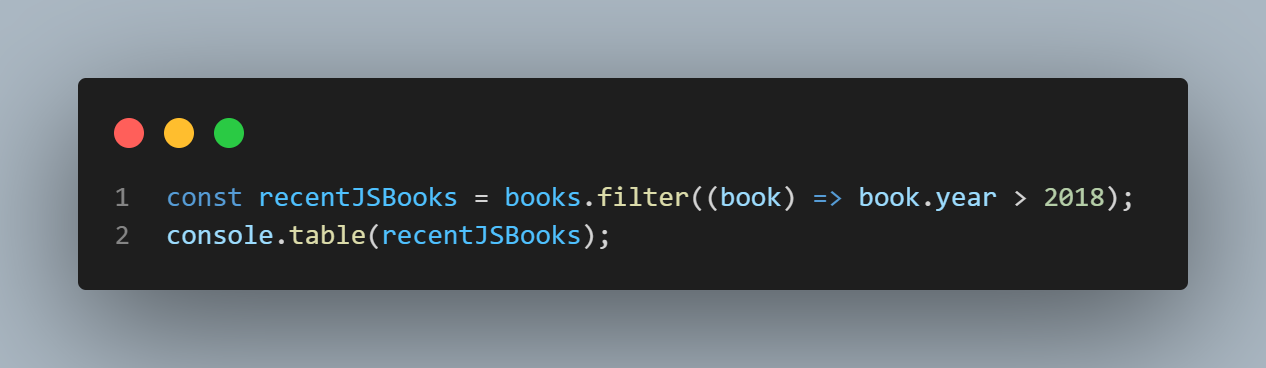
In addition, the following code snippet shows how to apply the map() method to the filtered data so that we can return textual descriptions of the recent JavaScript books.
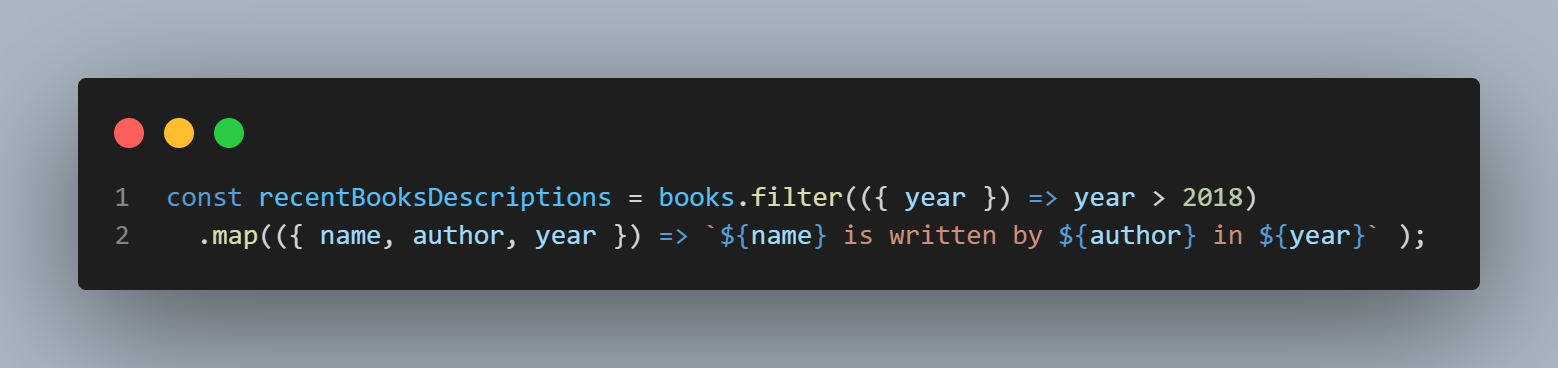
The reduce Method
As in map and filter, the reduce(callback) executes the given callback function (known as a reducer) on each element of the array, in order, while passing in the previously calculated value from the previous array element to the next element and returning a single value by the end. The following code snippet shows how to use the reduce method to calculate the sum of all elements in the array.
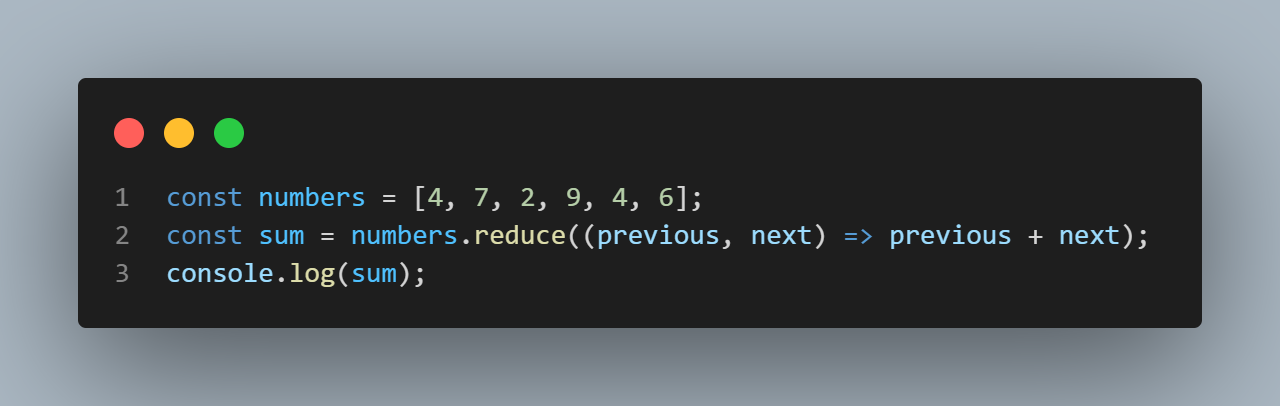
If you did not specify an initial value, the reduce method will use the element at index 0 as the initial value, and the calculation starts from the second element (element at index 1).