JavaScript Type System
JavaScript is known to be dynamically and weakly or loosely typed.
In any programming language, the type system is usually built into the compiler or interpreter to assign a type to a value or any programming construct (such as variables and functions) and specify the allowed operations on each data type. The type system is useful in several aspects, such as reducing type errors and enabling certain compiler optimizations. Programming languages can check for the type during compile time (static), run time (dynamic), or at both. Programming languages are classified based on the type checking (static vs dynamic) and the type safety (strong vs weak). So, let’s try to understand the difference between them.
Type Checking (When?): Static versus Dynamic
Type checking determines when types are checked and verified. For example, a dynamically-typed programming language checks for the types during run time. However, a statically-typed language checks the types and any syntactic errors during the compile time (before the actual running or execution of the code). JavaScript, PHP, Python and Ruby are examples of dynamically-typed languages. For example, in JavaScript, you can write:
let age = 20;
So, in the above statement, we have declared a variable without explicitly specifying the data type. The JavaScript interpreter assigns the proper type based on the given value (which will be a number in the above example) and checks for type errors during the run-time. On the other hand, Java, C, C++, Go and Scala are examples of statically-typed languages. Using a statically-typed langauage means that you must explicitly declare the type of the variable. For example, Java would throw a compile-time error if you forgot to specify the type in the following statement.
int age = 20;
Compile time type checking is static, while run time checking is dynamic. Static typing can help catch errors at compile time, and can also improve the performance of a program. Howver, a dynamically typed language is usually flexible and easier to use, but can result in more run time errors.
Type Safety (How Strict/Serious?): Weak versus Strong
Strongly typed programming languages have stricter typing rules, such as preventing the use of a variable in a way that is incompatible with its type. However, weakly typed languages have more flexible and relaxed typing rules. For example, in a strongly typed language, an explicit data type conversion may be required even if the implicit data type conversion does not cause any problems. Strong and weak typing is a somewhat relative spectrum and not fixed classes, so the typing system of a language may be stronger or weaker than the other. For example, PHP can be classified as a weakly typed language; however, it is more strongly typed than JavaScript. Moreover, strong and weak typing are not related to static and dynamic typing. For example, a dynamically typed language could be either strongly or weakly typed and vice versa. For example, Python is both dynamically- and strongly-typed, as shown in the following type system quadrant:
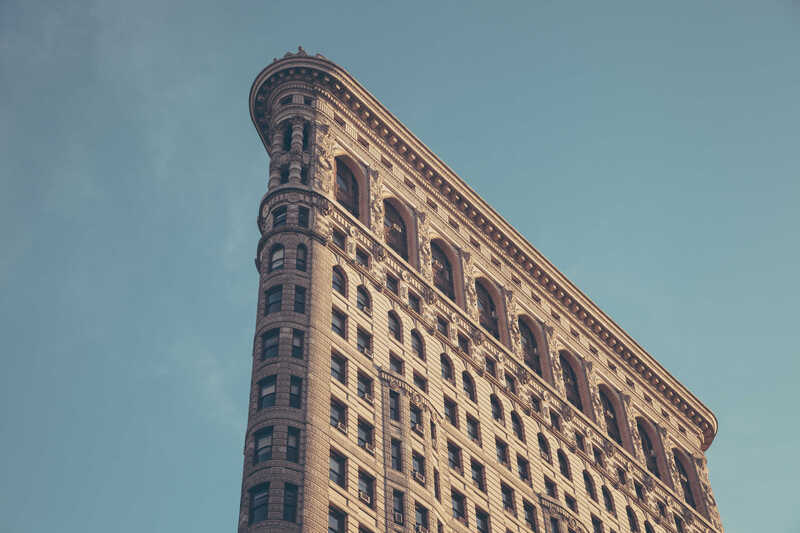
Strict? Strong, else weak.
Try the following code in JavaScript to understand how a weekly-typed language could behave:
1
2
3
4
5
6
console.log("7" + 3);
console.log("7" * 3);
console.log("7" / 3);
console.log(true / 3);
console.log(true + 3);
console.log(false + 3);
Test your Understanding
- Which of the following describes
static typing
?- A. Type checking is performed at run time
- B. Type checking is performed at compile time
- C. Type checking is performed at run and compile times
- Which of the following describes
dynamic typing
?- A. Type checking is performed at run time
- B. Type checking is performed at compile time
- C. Type checking is performed at run and compile times
- Which of the following describes
strong typing
?- A. Type checking is strict and will not allow operations between incompatible types without type conversion
- B. Type checking is lenient and will allow operations between incompatible types
- C. Type checking is always static in strong typing
- C. Type checking is always dynamic in weak typing
- Which of the following describes
weak typing
?- A. Type checking is strict and will not allow operations between incompatible types without conversion
- B. Type checking is lenient and will allow operations between incompatible types
- C. Type checking is always static in strong typing
- D. Type checking is always dynamic in weak typing
- Which of the following languages is
dynamically typed
?- A. Java
- B. JavaScript
- C. C++
- D. Go